In this lecture of how to design a chatbot we will at first make the HTML page.
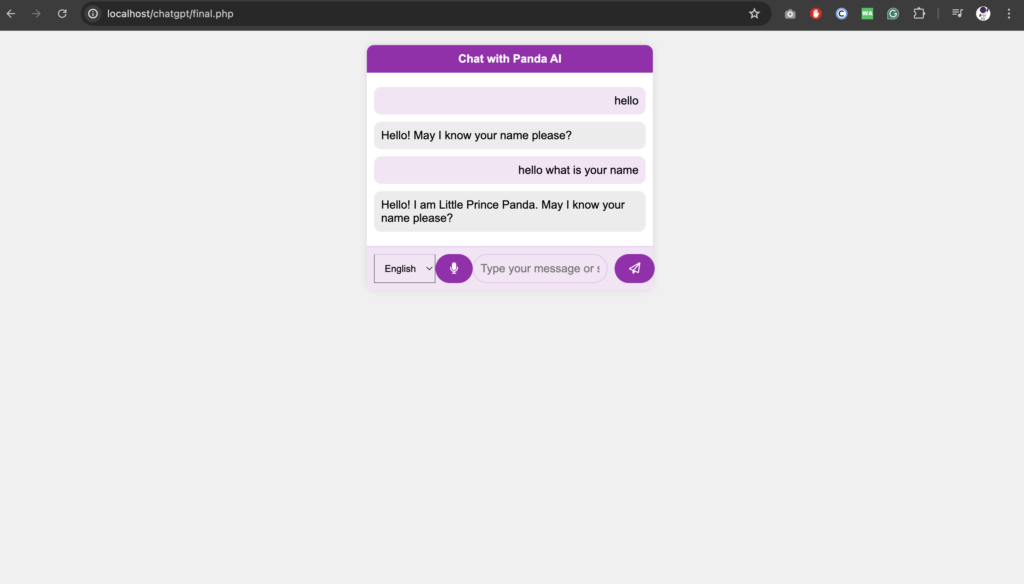
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chat with Panda AI</title>
</head>
<body>
<div>
<div>Chat with Panda AI</div>
<div>
<!--- Here We Will Add The Chat Messages -->
</div>
<form method="POST">
<button type="submit">Send</button>
</form>
</div>
</body>
</html>
We will now add style to the background and the chat container.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chat with Panda AI</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.chat-container {
max-width: 400px;
margin: 20px auto;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
</style>
</head>
<body>
<div class="chat-container">
<div>Chat with Panda AI</div>
<div>
<!--- Here We Will Add The Chat Messages -->
</div>
<form method="POST">
<button type="submit">Send</button>
</form>
</div>
</body>
</html>
Let us now add color to the chat header, add voice selection drop down and typing field
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chat with Panda AI</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css" integrity="sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.chat-container {
max-width: 400px;
margin: 20px auto;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.chat-header {
background-color: #9c27b0; /* Purple color */
color: #fff;
padding: 10px;
text-align: center;
font-weight: bold;
border-top-left-radius: 10px;
border-top-right-radius: 10px;
}
.chat-input {
display: flex;
padding: 10px;
background-color: #f3e5f5; /* Light purple color */
border-top: 1px solid #e1bee7; /* Border color */
}
.chat-input input {
flex-grow: 1;
padding: 10px;
border: 1px solid #e1bee7; /* Border color */
border-radius: 20px; /* Rounded corners */
margin-right: 10px;
outline: none; /* Remove default outline */
font-size: 16px; /* Font size */
}
.chat-input input:focus {
border-color: #9c27b0; /* Purple color when focused */
}
.chat-input button {
padding: 10px 20px;
background-color: #9c27b0; /* Purple color */
color: #fff;
border: none;
border-radius: 20px; /* Rounded corners */
cursor: pointer;
font-size: 16px; /* Font size */
outline: none; /* Remove default outline */
transition: background-color 0.3s; /* Transition effect */
}
.chat-input button:hover {
background-color: #7b1fa2; /* Darker purple color when hovered */
}
</style>
</head>
<body>
<div class="chat-container">
<div class="chat-header">Chat with Panda AI</div>
<div class="">
<!--- Here We Will Add The Chat Messages -->
</div>
<form class="chat-input" method="POST">
<select class="chat-input input">
<option value="en-US">English</option>
<option value="hi-IN">Hindi</option>
<!-- Add more options as needed -->
</select>
<input type="text" class="chat-input" name="userMessage" placeholder="Type your message or speak..." required>
<button type="submit"><i class="fa-regular fa-paper-plane"></i></button>
</form>
</div>
</body>
</html>
Final Design Of The ChatBot
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chat with Panda AI</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css" integrity="sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.chat-container {
max-width: 400px;
margin: 20px auto;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.chat-header {
background-color: #9c27b0; /* Purple color */
color: #fff;
padding: 10px;
text-align: center;
font-weight: bold;
border-top-left-radius: 10px;
border-top-right-radius: 10px;
}
.chat-messages {
padding: 10px;
max-height: 400px;
overflow-y: auto;
}
.message {
margin: 10px 0;
padding: 10px;
border-radius: 10px;
}
.message.user {
background-color: #f3e5f5; /* Light purple color */
text-align: right;
}
.message.assistant {
background-color: #ececec;
}
.chat-input {
display: flex;
padding: 10px;
background-color: #f3e5f5; /* Light purple color */
border-top: 1px solid #e1bee7; /* Border color */
}
.chat-input input {
flex-grow: 1;
padding: 10px;
border: 1px solid #e1bee7; /* Border color */
border-radius: 20px; /* Rounded corners */
margin-right: 10px;
outline: none; /* Remove default outline */
font-size: 16px; /* Font size */
}
.chat-input input:focus {
border-color: #9c27b0; /* Purple color when focused */
}
.chat-input button {
padding: 10px 20px;
background-color: #9c27b0; /* Purple color */
color: #fff;
border: none;
border-radius: 20px; /* Rounded corners */
cursor: pointer;
font-size: 16px; /* Font size */
outline: none; /* Remove default outline */
transition: background-color 0.3s; /* Transition effect */
}
.chat-input button:hover {
background-color: #7b1fa2; /* Darker purple color when hovered */
}
</style>
</head>
<body>
<div class="chat-container">
<div class="chat-header">Chat with Panda AI</div>
<div class="chat-messages">
<!--- Here We Will Add The Chat Messages -->
</div>
<form class="chat-input" method="POST">
<select id="language" class="chat-input input">
<option value="en-US">English</option>
<option value="hi-IN">Hindi</option>
<!-- Add more options as needed -->
</select>
<input type="text" class="chat-input" name="userMessage" placeholder="Type your message or speak..." required>
<button type="submit"><i class="fa-regular fa-paper-plane"></i></button>
</form>
</div>
</body>
</html>